In today’s fast-paced software development landscape, maintaining high-quality software is paramount. Automated testing and debugging play a critical role in ensuring that software meets the required standards of functionality, reliability, and performance. By incorporating automated processes, developers can detect and fix issues early in the development cycle, leading to more robust and dependable applications.
Understanding Automated Testing
Automated testing is the process of using software tools to execute pre-scripted tests on a software application before it is released into production. This method saves time and effort compared to manual testing, where testers need to perform each test step manually. Automated tests can be run repeatedly at any time of day, providing quick feedback to developers about the quality of their code.
The primary purpose of automated testing is to streamline the testing process, ensuring that the software behaves as expected under various conditions. It helps in identifying defects early, reducing the cost and effort required to fix them later in the development process. Additionally, automated testing supports continuous integration and continuous delivery (CI/CD) practices, enabling faster and more reliable software releases.
Types of Automated Tests
- Unit Testing:
- Definition: Focuses on individual components or units of the software, typically functions or methods.
- Purpose: Ensures that each unit performs as intended in isolation.
- Example Tools: JUnit, NUnit, pytest.
- Integration Testing:
- Definition: Verifies that different modules or services work together as expected.
- Purpose: Detects issues that occur when components interact.
- Example Tools: TestNG, FitNesse.
- System Testing:
- Definition: Examines the complete and integrated software system.
- Purpose: Ensures that the application functions correctly in its entirety.
- Example Tools: Selenium, QTP.
- Regression Testing:
- Definition: Re-runs previous tests to ensure that new code changes have not affected existing functionality.
- Purpose: Confirms that recent updates do not introduce new bugs.
- Example Tools: Jenkins, Bamboo.
Best Practices for Automated Testing
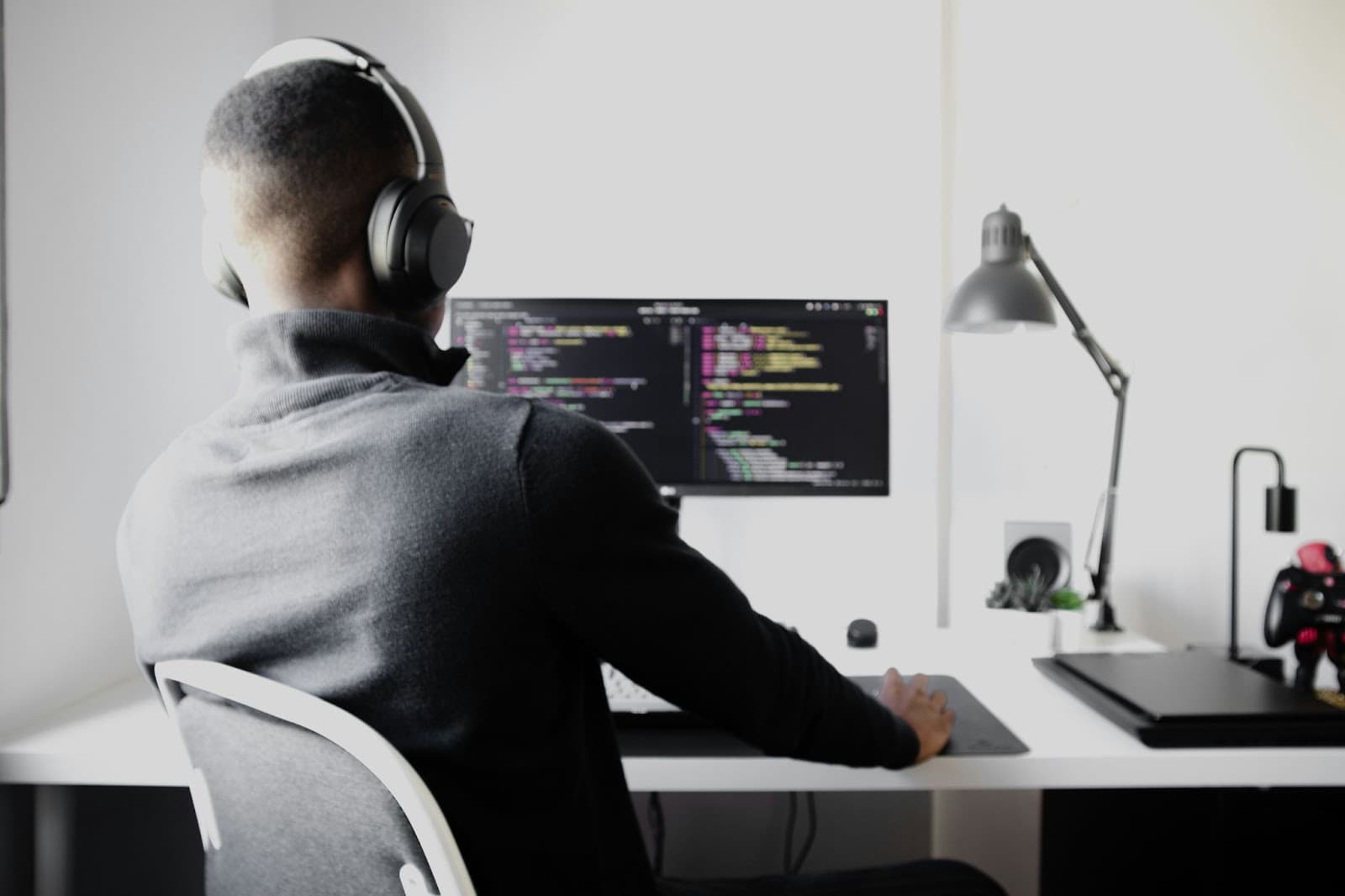
- Writing Clear and Maintainable Test Cases:
- Ensure that test cases are well-documented and easy to understand.
- Use descriptive names for tests and avoid hard-coding values.
- Organize tests logically, grouping similar tests together.
- Utilizing Continuous Integration (CI) Tools:
- Integrate automated tests into the CI pipeline to run tests automatically on every code commit.
- Use tools like Jenkins, CircleCI, or Travis CI to automate the execution of test suites.
- Prioritizing Tests Based on Risk and Impact:
- Identify and prioritize critical test cases that cover the most important functionality.
- Focus on tests that have the highest impact on the application’s stability and user experience.
- Regularly Reviewing and Refactoring Test Code:
- Conduct periodic reviews of test cases to identify and remove redundant or outdated tests.
- Refactor test code to improve readability, maintainability, and performance.
- Using Data-Driven and Behavior-Driven Development (BDD) Approaches:
- Implement data-driven testing to run tests with different sets of input data.
- Adopt BDD practices using frameworks like Cucumber to write tests in a human-readable format.
Common Tools for Automated Testing
- Selenium:
- Purpose: Web application testing.
- Key Features: Supports multiple browsers and operating systems, robust test automation capabilities, and integration with various programming languages (Java, C#, Python).
- Use Case: Ideal for functional and regression testing of web applications.
- JUnit:
- Purpose: Unit testing for Java applications.
- Key Features: Simple and efficient framework, extensive support for annotations, and integration with development environments like Eclipse and IntelliJ IDEA.
- Use Case: Essential for test-driven development (TDD) and ensuring code correctness at the unit level.
- TestNG:
- Purpose: Advanced testing framework inspired by JUnit.
- Key Features: Flexible test configuration, powerful execution model, support for parallel test execution, and data-driven testing.
- Use Case: Suitable for a wide range of testing needs, including unit, functional, end-to-end, and integration testing.
- Cypress:
- Purpose: Front-end testing for web applications.
- Key Features: Real-time reloading, automatic waiting, and built-in support for modern JavaScript frameworks.
- Use Case: Excellent for end-to-end testing and debugging of modern web applications.
- Jenkins:
- Purpose: Continuous integration and continuous delivery (CI/CD) tool.
- Key Features: Automation of the build and deployment process, extensive plugin ecosystem, and integration with various version control systems.
- Use Case: Automates running automated tests as part of the CI/CD pipeline, ensuring consistent and reliable code quality.
Effective Debugging Strategies
- Systematic Approach:
- Steps: Identify the problem, isolate the issue, find the root cause, and apply the fix.
- Tip: Break down the problem into smaller, manageable parts to identify the faulty component.
- Using Logging and Monitoring Tools:
- Tools: Log4j, Splunk, ELK Stack (Elasticsearch, Logstash, Kibana).
- Tip: Implement comprehensive logging and monitor application behavior to quickly identify issues.
- Leveraging Debugging Tools:
- Tools: GDB (GNU Debugger), Visual Studio Debugger, Chrome DevTools.
- Tip: Use breakpoints, watch expressions, and step-through execution to pinpoint the exact location of the issue.
- Clear and Descriptive Error Messages:
- Tip: Ensure error messages provide enough context to understand what went wrong and where.
- Example: Instead of “NullPointerException,” provide “NullPointerException in Class X at Method Y due to Variable Z.”
- Collaborating with Team Members:
- Tip: Pair programming and code reviews can help spot issues that might be overlooked by individual developers.
- Benefit: Leverages collective expertise and fosters a collaborative debugging process.
Integrating Automated Testing and Debugging in the Development Workflow
- Setting Up a Robust CI/CD Pipeline:
- Action: Integrate automated tests into the CI/CD pipeline using tools like Jenkins, CircleCI, or GitLab CI.
- Benefit: Ensures tests are run automatically on every code commit, providing immediate feedback on code quality.
- Regular Automated Test Runs and Immediate Bug Fixes:
- Action: Schedule regular test runs and prioritize fixing any issues that arise.
- Benefit: Keeps the codebase clean and reduces the risk of introducing new bugs.
- Keeping Test Suites Up-to-Date with Code Changes:
- Action: Update test cases and scripts to reflect new features or changes in the codebase.
- Benefit: Ensures that tests remain relevant and effective in detecting issues.
- Encouraging a Culture of Testing and Quality Assurance:
- Action: Promote best practices in testing and debugging through training, code reviews, and collaboration.
- Benefit: Fosters a quality-first mindset among team members, leading to more reliable software.
Future Trends in Automated Testing and Debugging
- AI and Machine Learning in Testing:
- Overview: AI and machine learning are transforming automated testing by enabling smarter test case generation, predictive analytics, and automated defect detection.
- Benefits: AI-driven testing tools can identify patterns, predict potential failures, and optimize test coverage, leading to more efficient and effective testing processes.
- Example Tools: Applitools (visual AI testing), Testim.io (AI-based test automation).
- Shift-Left Testing:
- Overview: The shift-left approach involves integrating testing early in the software development lifecycle (SDLC) to detect and fix defects sooner.
- Benefits: Early detection of issues reduces the cost and effort of bug fixes, improves software quality, and accelerates the development process.
- Implementation: Incorporate unit and integration testing in the initial development phases, utilize CI/CD pipelines for continuous testing.
- Continuous Testing:
- Overview: Continuous testing involves running automated tests at every stage of the CI/CD pipeline to ensure ongoing code quality and compliance.
- Benefits: Provides real-time feedback on software health, supports rapid release cycles, and ensures consistent quality across releases.
- Example Tools: Jenkins, GitLab CI/CD, CircleCI.
- Test Automation for Microservices and Containerized Applications:
- Overview: With the rise of microservices and containerized applications, automated testing tools are evolving to handle the complexities of these architectures.
- Benefits: Enhanced scalability, flexibility, and reliability in testing distributed systems.
- Example Tools: Docker, Kubernetes, Istio for service mesh testing.
- Enhanced Debugging with Observability:
- Overview: Observability combines logging, monitoring, and tracing to provide a comprehensive view of system behavior, aiding in effective debugging.
- Benefits: Helps identify and resolve issues quickly by providing deep insights into system performance and anomalies.
- Example Tools: Prometheus (monitoring), Grafana (visualization), Jaeger (tracing).
Final Thoughts
Automated testing and debugging are essential components of modern software development, ensuring that applications meet high standards of quality and reliability. By leveraging advanced tools and best practices, developers can streamline their workflows, reduce the time and effort required to identify and fix issues, and deliver robust software products.
The future of automated testing and debugging is bright, with emerging trends like AI-driven testing, shift-left approaches, continuous testing, and enhanced observability set to further transform the landscape. By staying abreast of these trends and continuously improving their testing and debugging strategies, development teams can achieve greater efficiency, faster release cycles, and higher software quality.
Implementing a robust automated testing and debugging framework not only enhances software quality but also fosters a culture of continuous improvement and innovation, ultimately driving success in the competitive world of software development.